파일 입출력
파일 입출력을 사용하는 이유
- 파일로부터 데이터를 읽어와서 프로그램에 사용하기 위해
- 프로그램에서 만든 데이터를 파일형태로 저장하기 위해
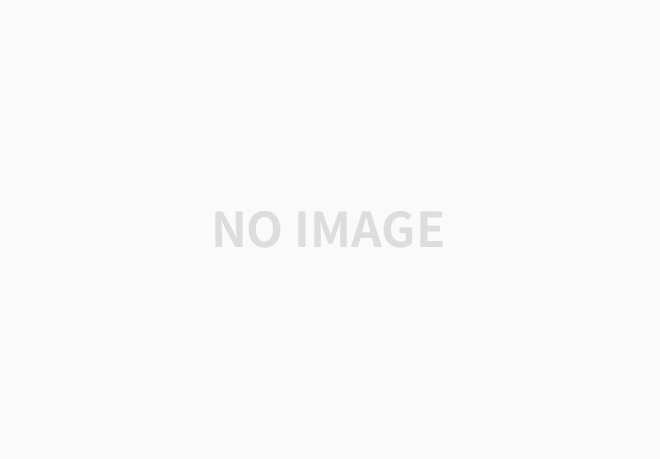
파일 열기 모드
- w: 쓰기 모드 (write)
- a: 추가 모드 (append)
- r: 읽기 모드 (read)
파일 쓰기 -> 덮어쓰기
파일객체 = open("파일이름", "파일모드")
파일객체.write(data)
파일객체.close()
ex)
file = open("data.txt", "w")
file.write("write")
file.close()
파일 추가 -> 이어쓰기
파일객체 = open("파일이름", "파일모드")
파일객체.write(data)
파일객체.close()
ex)
file = open("data.txt", "a")
file.write("append")
file.close()
파일 읽기
파일객체 = open("파일이름", "파일모드")
파일객체.read()
파일객체.close()
ex)
file = open("data.txt", "r")
file.write("append")
file.close()
파일 한 줄 읽기
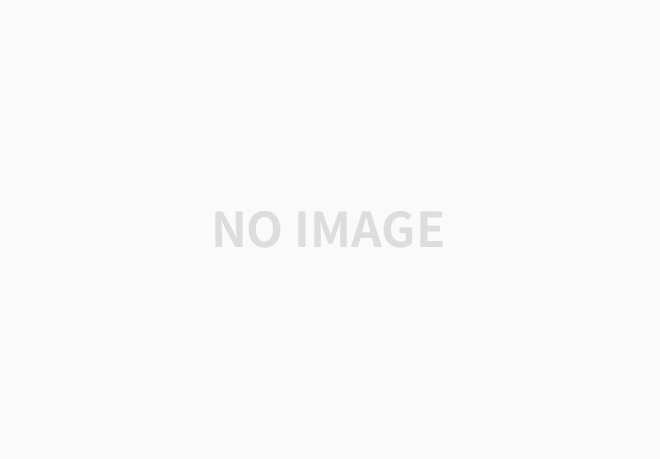
pickle 모듈
파일에 파이썬 객체를 저장하기 위해 사용되는 모듈
파일에 파이썬 객체 저장하기
import pickle
data = { ... }
file = open("data.pickle", "wb") //wb는 binary형태의 write. 컴퓨터가 바로 읽을 수 있게끔
pickle.dump(data,file) //위의 data.pickle에 data가 저장됨
file.close
파일로부터 파이썬 객체 읽기
import pickle
file = open("data.pickle", "rb")
data=pickle.load(file)
print(data)
file.close()
with 구문
- with 구문 사용 x
file = open("data.txt","r")
data = file.read()
file.close()
- with 구문 사용 O (file.close()를 계속 사용하는 것이 번거로워서)
with open("data.txt","r") as file:
data = file.read()
csv 파일
csv 파일이란?
csv (comma-separated values): 데이터가 콤마로 구분된 텍스트 파일 형식
-> 표 형태의 데이터!
csv 파일 쓰기
file = open ("student.csv", "w")
writer =csv.writer(file)
for d in data:
writer.writerow(d)
file.close()
csv 파일 읽기
import csv
file = open("student.csv", "r")
reader = csv.reader(file)
for d in reader:
print(d)
file.close()
예외처리
예외처리가 필요한 이유
프로그램 실행 중에 발생하는 에러를 미연에 방지하기 위한 것
try - except 구문
try:
예외가 발생할 수 있는 코드
except:
예외 발생 시 실행할 코드
else, finally
else:
예외 발생하지 않은 경우 실행할 코드
finally:
항상 실행할 코드
raise 구문 사용법
-> raise는 에러를 강제로 발생시키기 위해 사용
raise 예외("에러메세지")
ex) raise Exception
raise Exception("에러메시지")
예외 계층 구조
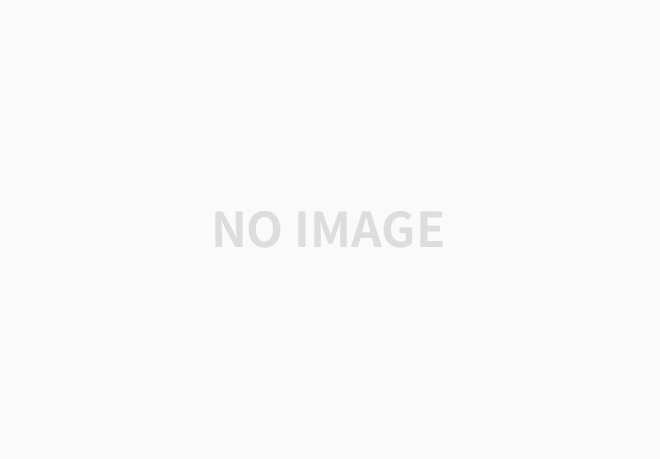
에러 만들기
class 예외 (Exception):
def__init__(self):
super().__init__("에러 메세지")
'Python' 카테고리의 다른 글
(8)Python: 모듈, 패키지 (0) | 2022.01.25 |
---|---|
(7)Python: 클래스와 객체, 생성자, 상속, 오버라이딩 (0) | 2022.01.14 |
(6) Python: 함수, 튜플, 딕셔너리 (0) | 2022.01.13 |
(5) Python: 제어문 (조건문, 리스트, 반복문) (0) | 2022.01.11 |
(4) Python: 연산, 데이터 입력, 자료형 변환 (0) | 2021.08.26 |